We will learn how to write the data into a temporary file in java using File class.
Below is the example program.
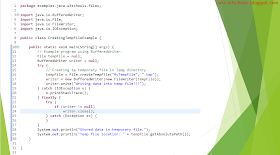
Below is the example program.
Writing data into Temp File:
package examples.java.w3schools.files; import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException; public class CreatingTempFileExample { public static void main(String[] args) { // Example program using BufferedWriter. File tempFile = null; BufferedWriter writer = null; try { // Creating ta temporary file in Temp directory. tempFile = File.createTempFile("MyTempFile", ".tmp"); writer = new BufferedWriter(new FileWriter(tempFile)); writer.write("Writing data into temp file!!!. This is a temp file."); } catch (IOException e) { e.printStackTrace(); } finally { try { if (writer != null) writer.close(); } catch (Exception ex) { } } System.out.println("Stored data in temporary file."); System.out.println("Temp file location: " + tempFile.getAbsolutePath()); } }
Output:
The above program produces the following output and executed in windows operating system.
Stored data in temporary file. Temp file location: C:\Users\user\AppData\Local\Temp\1\MyTempFile3113093780191713532.tmp
Temporary file is stored in the users Temp location. You can open the file and see its content.
No comments:
Post a Comment
Please do not add any spam links in the comments section.